How to include Facebook Android SDK in project as maven module
This post will be usefull if you have Android maven multimodule project and want to include Facebook Android SDK in your project as module.
How do this?
As you see here https://github.com/facebook/facebook-android-sdk the Facebook SDK used not as maven module.
Below you can download source code of Android project
To do this you need:
1. Download the Facebook Android SDK from github.
2. Add Facebook Android SDK lib project in your workspace near other modules of your maven project. Look at screenshot below:
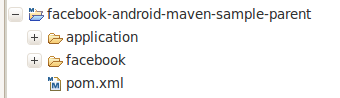
3. Ensure that your facebook dir contauns next files and dirs. Look at screenshot below:
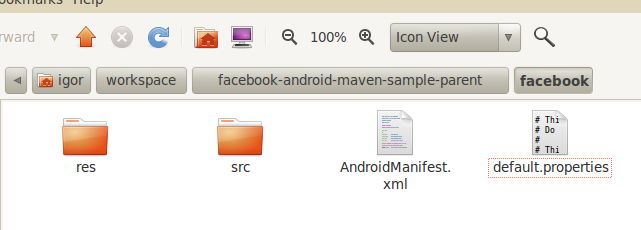
4. Add to parent project’s pom.xml new module. The
<modules>
<module>facebook</module>
<module>application</module>
</modules>
5. Add in to facebook dir next pom.xml file. Don’t forget setup group_id, artifact_id and version of your parent project. I noticed in code where you need to do this:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemalocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelversion>4.0.0</modelversion>
</project>
<parent>
<groupid>YOUR_PARENT_PROJECT_GROUP_ID</groupid>
<artifactid>YOUR_PARENT_PROJECT_ARTIFACT_ID</artifactid>
<version>YOUR_PARENT_PROJECT_VERSION</version>
</parent>
<groupid>com.facebook.android</groupid>
<artifactid>facebook-android-sdk</artifactid>
<version>0.0.1</version>
<packaging>jar</packaging>
<name>Facebook Android SDK</name>
<dependencies>
<dependency>
<groupid>com.google.android</groupid>
<artifactid>android</artifactid>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupid>com.jayway.maven.plugins.android.generation2</groupid>
<artifactid>maven-android-plugin</artifactid>
<configuration>
<androidmanifestfile>${project.basedir}/AndroidManifest.xml
</androidmanifestfile>
<assetsdirectory>${project.basedir}/assets</assetsdirectory>
<resourcedirectory>${project.basedir}/res</resourcedirectory>
<nativelibrariesdirectory>${project.basedir}/src/main/native
</nativelibrariesdirectory>
<sdk>
<platform>8</platform>
</sdk>
<deleteconflictingfiles>true</deleteconflictingfiles>
<undeploybeforedeploy>true</undeploybeforedeploy>
</configuration>
<extensions>true</extensions>
</plugin>
<plugin>
<artifactid>maven-compiler-plugin</artifactid>
<configuration>
<source>1.6</source>
<target>1.6</target>
</configuration>
</plugin>
</plugins>
</build>
6. Change path to java source files from src/com/facebook/android to src/main/java/com/facebook/android
7. Delete all projects from your Eclipse’s package explorer.
8. Import project as Maven project. If you do it right you can see next:
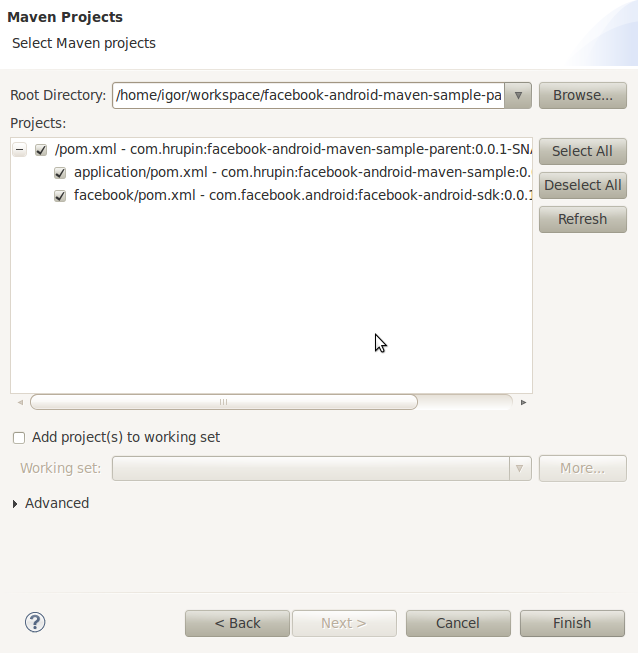
When you import this project the Eclipse will create target dir in your facebook project with APK file.
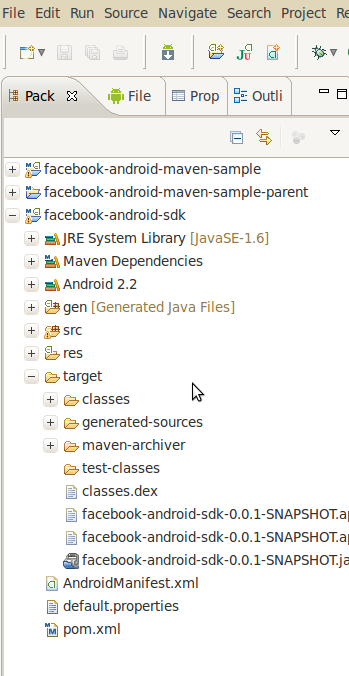
8. Add Facebook Android SDK dependencies in your application. To do this open pom.xml of your app and add next code in <dependencies>.
<dependency>
<groupid>com.facebook.android</groupid>
<artifactid>facebook-android-sdk</artifactid>
<version>0.0.1</version>
</dependency>
And whole pom.xml of your app need to be looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemalocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelversion>4.0.0</modelversion>
</project>
<parent>
<groupid>YOUR_PARENT_PROJECT_GROUP_ID</groupid>
<artifactid>YOUR_PARENT_PROJECT_ARTIFACT_ID</artifactid>
<version>YOUR_PARENT_PROJECT_VERSION</version>
</parent>
<groupid>com.hrupin</groupid>
<artifactid>facebook-android-maven-sample</artifactid>
<version>0.0.1-SNAPSHOT</version>
<packaging>apk</packaging>
<name>facebook-android-maven-sample - Application</name>
<dependencies>
<dependency>
<groupid>com.google.android</groupid>
<artifactid>android</artifactid>
</dependency>
<dependency>
<groupid>com.facebook.android</groupid>
<artifactid>facebook-android-sdk</artifactid>
<version>0.0.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupid>com.jayway.maven.plugins.android.generation2</groupid>
<artifactid>maven-android-plugin</artifactid>
<configuration>
<androidmanifestfile>${project.basedir}/AndroidManifest.xml</androidmanifestfile>
<assetsdirectory>${project.basedir}/assets</assetsdirectory>
<resourcedirectory>${project.basedir}/res</resourcedirectory>
<nativelibrariesdirectory>${project.basedir}/src/main/native</nativelibrariesdirectory>
<sdk>
<platform>8</platform>
</sdk>
<deleteconflictingfiles>true</deleteconflictingfiles>
<undeploybeforedeploy>true</undeploybeforedeploy>
</configuration>
<extensions>true</extensions>
</plugin>
<plugin>
<artifactid>maven-compiler-plugin</artifactid>
<configuration>
<source />1.6
<target>1.6</target>
</configuration>
</plugin>
</plugins>
</build>
That’s all. Try to build your project with maven:
cd your_parent_project_dir
mvn clean install
This sample is not detailed. If you have some troubles you can leave me comment and i will try to ask you.
Hope, it help you!
Here you can download sample project with Facebook Android SDK as maven module

1 Comment
Elbert Rob · 13 November, 2011 at 14:20
I really like to read this post but I don’t have Android maven multimodule project. I think it’ll be very helpful those who are working with this project. Great post! Thanks 🙂
Comments are closed.